APIs can be tough to get into, but fast API makes it pretty simple.
For example lets say I want to make an API call to build a basic DND char. It’s actually pretty durn easy:
The code to build an API endpoint
# This file will be called build_char.py
from fastapi import FastAPI #Import Fast API
import random
app = FastAPI() # Define a fast API instance
#Random code to make a DnD NPC
races = ["Human", "Elf", "Dwarf", "Halfling", "Gnome", "Half-Elf", "Half-Orc", "Dragonborn"]
jobs = ["Barkeep", "Blacksmith", "Bounty Hunter", "Burglar", "Clergy", "Farmer", "Guard", "Merchant", "Noble", "Thief"]
def generate_npc():
race = random.choice(races)
job = random.choice(jobs)
alignment = random.choice(["Lawful Good", "Neutral Good", "Chaotic Good", "Lawful Neutral", "True Neutral", "Chaotic Neutral", "Lawful Evil", "Neutral Evil", "Chaotic Evil"])
strength = random.randint(1, 20)
dexterity = random.randint(1, 20)
intelligence = random.randint(1, 20)
wisdom = random.randint(1, 20)
charisma = random.randint(1, 20)
return ( race, job, alignment, strength, dexterity, intelligence, wisdom, charisma)
# This is creating the endpoint /generate_npc, and running the function that is tied to that end point when the code is run.
@app.get("/generate_npc")
def generate_npc_endpoint():
npc = generate_npc()
return npc
How to make that code callable
From your CLI in the same folder as the code above you’ll run
python -m uvicorn build_char:app –reload
With the build_char being the name of the file it’s looking for, and app being the name of the FastAPI instance, also the @app that was referenced in the decorator where we built our end point. So lets make that API call to http://127.0.0.1:8000/generate_npc
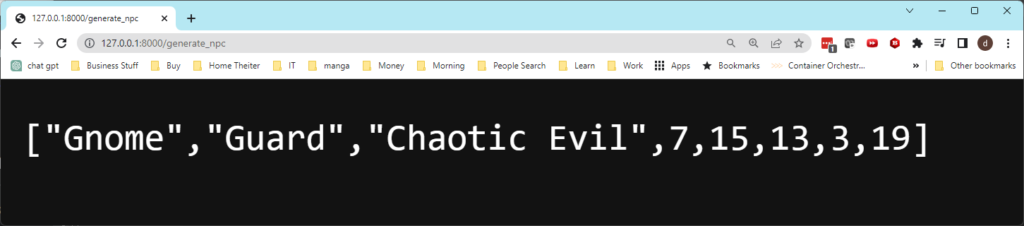
As you can see we now have the output of the function accessible via an API call.